Scope of variables in python
In Python programming, declare variable scope determines the access nature of the variable in the current function program, that is how any declare program variable local or global variable name can be accessed in your current function program code. Determining the current program variable scope is necessary to write any Python program bug-free and maintainable program code.
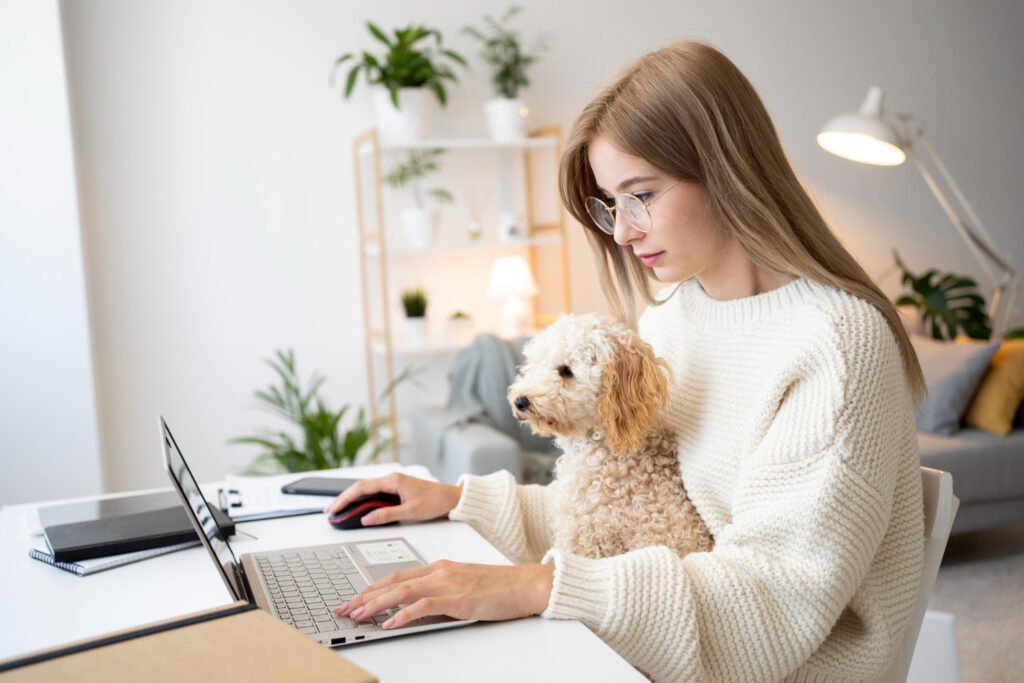
So let’s understand the declare parameter variable scope in Python function program better.
Python Local Variable Scope.
Function parameter/argument variables declared inside any Python program function are declared as local variable scope of that function. Remember that local function variables are called and accessed only inside that function.
Python Local Variable Scope Example.
def local_function(p):
p = 7 # declare Local parameter variable
print(f”local function declare Inside function – {p}”)
print(local_function)
print(p)
Here local_function() # result is – 7.
# print(p) # This will give an error because p is not declared/defined outside the function
In the above program example, p is a local nature variable inside local_function(). Remember that the p parameter can be accessed only inside the function, and if you try to print the p value outside the function scope, the program will give an error because here p parameter is not defined as a global variable.
Python Global Variable Scope.
Variables declared as global inside a function parameter declared/defined outside a function in the current Python program are of global scope nature. Remember that Python programs declare global functions which can be accessed and processed anywhere in the entire program including inside the function.
Python Global Variable Scope Example.
p = 7 # declare global scope parameter variable
def global_function():
print(f”local function – {p}”)
global_function() # the result is -local function – 7
print(f”global function – {p}”) # the result is – global function – 7
In the above function example, p is a global variable because it is declared/defined outside any function. It can be accessed from both inside and outside global_function() and the existing function program.
Modifying global variables in Python programs.
If you want to modify the global variable argument value inside an existing Python function. Then you have to use the global keyword to declare the modified parameter in the program that you want to modify the global variable.
Modifying global variables Example.
p = 1 # python function Global variable declare
def alter_globalvar():
global p
p = 2
print(f”global function Before call – {p}”) # the result is – global function Before call – 1
alter_globalvar()
print(f”global function After value modify – {p}”) # the result is – global function After value modify – 2
In the global function example above, the alter_globalvar() function modifies the value of the global variable p using the global p statement. This allows modifications made to p inside the function to affect the global scope.
Python nonlocal scope.
Variables in nested functions in a Python program can be declared in a scope called nonlocal. This is declared in a Python program when a variable is defined in the outer function, and a new value is assigned to it in the inner function. Which is defined with the nonlocal keyword.