Using break and continue statements in python
The break and continue statements in Python programming are the control flow statements in the program. They help the Python programmer to control the behavior of the program loop (for and while loop) based on some specific conditions like break and continue.
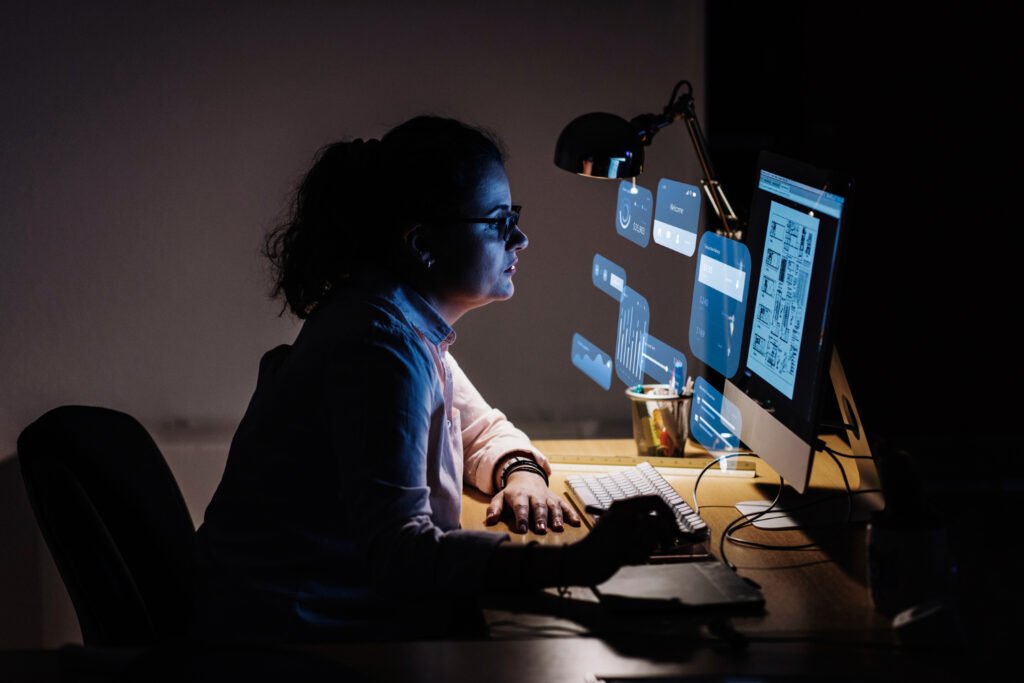
So let’s see how break and continue statement is used in Python programming.
Python break statement.
The break statement is used to break the Python program for and while loop prematurely, regardless of whether the condition of the current program loop is false or not. When you use the break statement in a Python program loop, it will break the program immediately, and it immediately exits the current program loop, and similarly the program continues to check the next program statement outside the loop. Until the loop condition is tested.
Example of use of for loop with break statements.
laptops = [“hp”, “dell”, “macbook”, “acer”, “lenovo”,”microsoft surface”]
for laptop in laptops:
print(laptop)
if laptop == “acer”:
break
In the above example.
Here laptops list element is repeated in laptops list.
It processes and prints each laptops list element.
When laptops variable reaches “acer”, then if condition laptop == “acer” becomes true.
And the break statement in the program gets executed, which terminates the loop immediately after printing laptops items upto “acer” element in laptops list.
Example of using break statement in while loop.
integer = 3
while integer <=15:
print(integer)
if integer == 9:
break
integer += 1
In the above while loop break statement example.
In this program the while loop continues as long as the integer value is less than 9.
It prints the current value of the integer number.
When the integer variable reaches the value 9, the if condition integer == 9 becomes true.
And the break statement is executed, which terminates the loop immediately after printing the decimal number 9.
Python continue Statement.
The continue statement in Python programs is used to skip a particular program code inside the loop for the current program repetition and proceed to the next repetition element part of the loop.
Example of using the continue statement in a for loop.
laptops = [“hp”, “dell”, “macbook”, “acer”, “lenovo”,”microsoft surface”]
for laptop in laptops:
print(laptop)
if laptop == “dell”:
continue
In the above Python continue program example.
Here the for loop iterates over the list laptops.
It checks if the list element laptops is “dell” or not.
If the list element laptops is “dell”, then the continue statement is executed.
Here the rest of the code inside the for loop (print(laptop)) is skipped, and the loop proceeds to the next iteration list element on the screen.
Example of using the continue statement in a while loop.
number = 0
while number <= 15:
number += 2
if number == 8:
continue
print(number)
In the above continue while program statement example.
As long as number is less than 8, the while loop continues.
Where number is incremented inside the for loop (number += 2).
When number becomes 8, the if condition number == 8 becomes true.
Here the continue statement gets executed when number is equal to 8 and the rest of the code (print(number)) of the loop is skipped.
And the loop proceeds with the next repetition point in the program.
Example of break and continue in for and while loop in Python.
break statement – In Python programs for loop and while loop, break statement is used to exit the program loop when a particular condition is met, whether the program loop terminates naturally or not.
continue statement – The continue statement is used to ignore some repetition part of the program loop based on a program condition, so that the program loop proceeds with the next list element repetition.
Both the for and while loops in Python programming are used to control the flow of program execution in a loop. They allow you to change the behavior of your program code based on particular conditions and requirements using break and continue statements.