Typedef in c
typedef is a reserved keyword in C programming. The typedef keyword is used to create data type aliases for existing data types or to create new named data types. It can simplify program declaration of complex data types, make program code more readable, and make program data types abstract.
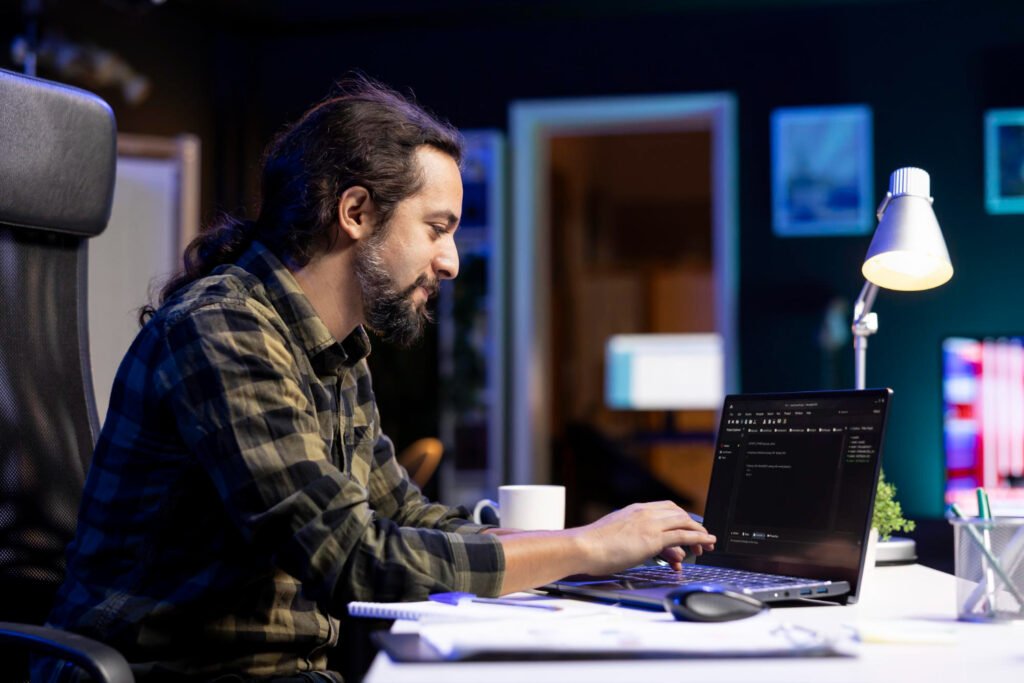
Basic use of typedef data type in C programs.
The typedef keyword in C programs allows you to define a new name for an existing data type. It can be especially useful for defining complex data types or making program code more readable.
typedef program examples in C.
#include <stdio.h>
typedef unsigned int number;
int main() {
number num = 78;
printf(“\n num is : %u”, num);
return 0;
}
In the above C program example, Number is defined as an alias for unsigned int.
Using typedef data type with structures in a program.
Typedef data types are often used in conjunction with structure data types to avoid repetitive and long type declarations in a typedef program.
C program example without typedef.
#include <stdio.h>
struct Values {
int p;
int q;
};
int main() {
struct Values m1;
m1.p = 1;
m1.q = 2;
printf(“\n The Values is – (%d, %d)\n”, m1.p, m1.q);
return 0;
}
C program example with typedef.
#include <stdio.h>
typedef struct {
int p;
int q;
} Values;
int main() {
Values m1;
m1.p = 1;
m1.q = 2;
printf(“\n the values with structure – (%d, %d)\n”, m1.p, m1.q);
return 0;
}
In the above C program example, values are defined as aliases for struct { int p; int q; }.
Using typedef data types with pointers.
typedef data types can be used to create alias data types for pointer types, making the program code more readable.
Example of typedef data types with pointers.
#include <stdio.h>
typedef int* int_pointer;
int main() {
int q = 9;
int_pointer p = &q;
printf(“\n the pointer values is – %d”, *p);
return 0;
}
Here, int_pointer is defined as an alias for int*.
Using typedef data types with pointers.
Declaring and using function pointers in a C program can be complex. Here typedef data types make this process easier.
Example of typedef data types with pointers.
#include <stdio.h>
typedef void (*function_pointer)(int);
void printInteger(int inte) {
printf(“\n the pointer is – %d”, inte);
}
int main() {
function_pointer fp = printInteger;
fp(9);
return 0;
}
In this function pointer example, function_pointer is defined as an alias for void (*)(int).
Using typedef data types with Arrays in C.
typedef can also be used to create aliases for array types.
Using typedef data types with Arrays in C.
#include <stdio.h>
typedef int IntegerArray[7];
int main() {
IntegerArray arry = {1, 2, 3, 4, 5, 6, 7};
for (int p = 0; p < 7; p++) {
printf(“\n the array value is – %d “, arry[p]);
}
printf(“\n”);
return 0;
}
Here, IntegerArray is defined as an alias for int[7].
Using typedef data types with enums.
typedef data types can be used to create aliases for enumeration data types, making program source code more readable.
Example of typedef with enums.
#include <stdio.h>
typedef enum {
YES,
NO,
INVALID
} Choice;
int main() {
Choice ch = YES;
printf(“\n the choice is – %d\n”, ch);
return 0;
}
In this enum to typedef program example, Choice is defined as an alias data type for enum { YES, NO, INVALID }.
Combining typedef with structures and enums in C programs.
You can combine typedef with structures and enums to create more complex type definitions.
Use of typedef data type in C.
In C programming, typedef data type is used to create alias data types for existing data types. It is a powerful tool for simplifying complex typedef declarations and making program code more readable.
Some key points about typedef.
Typedef creates type aliases for primitive data types in C programs, such as structures, pointers, function pointers, arrays, and enums
Typedef data types simplify the declaration of complex program types.
Typedef makes program source code readable and improves program maintainability.
Using typedef data types effectively helps you create clearer and more maintainable C program code.