Return values
In C programming functions can return argument values to the function calling source code, so that the function can calculate the argument results, and pass them back to the function calling for further function use or processing. So let’s see how you can declare a function that returns function argument values, use function definitions, and function callings in C programs.
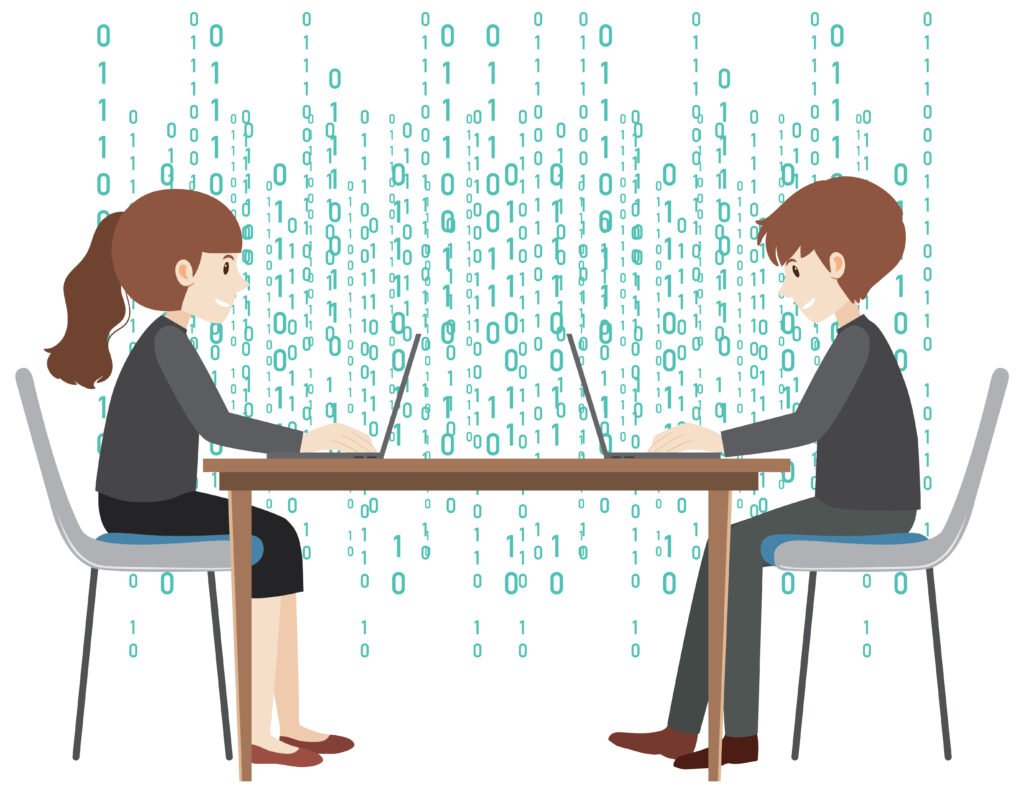
Function Declaration in C.
When a function is declared in a C program that returns a value function, you define the data type of the function value being returned. If the function you declare does not return any value, you use void() as the function return type.
Function declaration syntax.
return_type function_name(parameter_list);
return_type – Defines the data type of the returned value of the function you declare. It can be any valid C data type, including primitive data types (int, float, char, etc.) or user-defined data types (structures, pointers, etc.).
function_name – The name of the function declared by the programmer in a C program, which is a valid C program identifier.
parameter_list – The list of parameter (argument) variables accepted by the function. Each parameter has a data type and function parameter name separated by a comma operator.
function declaration Example.
// Function declaration
int total(int p, int q);
void display(char emp_name[]);
In the above example.
total is a function that returns an int value and takes two int variable argument parameters (p and q) as input.
display is a user-defined function. which returns void (no return value), and takes a single parameter, a char array (emp_name[]) array value.
Function Definition.
The function definition describes the actual function expression condition of the function being executed, which contains all the function calculations or function operations that it performs. This terminates by returning a function value using the return statement when applied to a function.
Function Definition Syntax.
return_type function_name(args/parameter_list) {
// function body (statements)
return expression; // Return function statement
}
return_type – Here you define the data type of the function value being returned by the function.
function_name – The name of your declared function, matching the declared function.
parameter_list – The list of function parameters with their data types and variable names, matching the function declaration.
expression – The expression is the value returned by the function. It can be a literal value, a variable, or a complex function expression.
Function definition syntax.
// Function definition for the total function
int total(int p, int q) {
int total = p + q;
return total; // Return the total of p and q
}
// Function definition for the hello function (no return value)
void hello(char stu_name[]) {
printf(“\n Hi, %s!”, stu_name);
Calling a declared function and using the return value in a C program.
To call a function that does not return a value in a function program, you usually assign the returned function value to a variable of the appropriate type, or use it directly in a program expression.
Calling function example.
int main() {
int output = total(1, 2); // Calling the total function and storing the result
printf(“\n the output of total – %d”, output);
hello(“welcome to c”); // Calling the hello function
return 0;
}
Important Points of Function.
Returning from main() – The main() function built-in in C language also returns an integer value to the operating system or the calling process. While by convention, a return value of 0 from main() is zero, a non-zero value usually indicates an error or abnormal program termination.
Multiple return statements – A user-declared function can have multiple return statements or parameters, each of which conditionally returns a different program function argument value depending on the program logic.
Return Type Compatibility – The data type of the value returned by the function must match the return type declared in its prototype and definition.
Examples of multiple return statements in a function.
// lets use Function to find the max of two integers
int large(int p, int q) {
if (p > q) {
return p;
} else {
return q;
}
}
int main() {
int s = 1, t = 2;
int large_number = large(s, t);
printf(“\n The largest number values is – %d and %d is: %d”, s, t, large_number);
return 0;
}
In this example, the large() function returns the larger integer value of two integers p and q using the conditional if statement return statement.
Understanding how to declare, function definition, and use value returning functions in C is essential to write modular and reusable program code in the C language. It allows you to contain arguments in a function.