Declaration and definition
Functions play an important role in C programming in organizing program code into smaller blocks for managing and reusing it and in calling individual program arguments. Functions are used as an alternative to modular programming in C programming. Where a large program project is declared in small modules or function blocks and called separately. Where functions make a large C program simpler, program maintenance easy, and convenient. How to declare a function in C program and how to define function arguments.
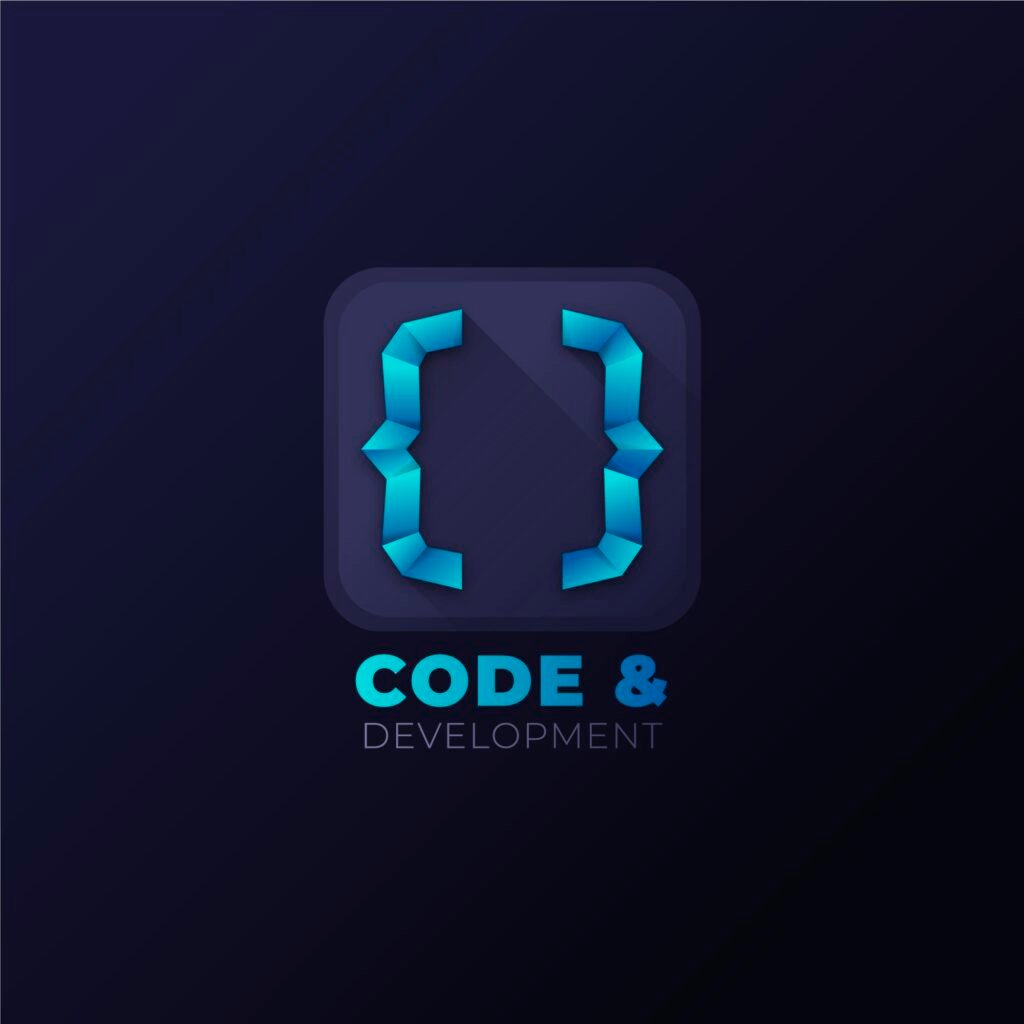
So, let’s declare a function in C language
Function declaration in C language.
How to declare a function in C program, first declare the function name, the return type of the function, the function calling parameter list (if any), and optionally, define any function modifier elements or objects. Here the function declaration tells the compiler at program execution time about the existence of the program function and how it should be called in the current program.
Function declaration syntax.
return_type function_name(argumentparameter_list);
return_type – The return type of the function defines the data type of the argument values to be returned by the program. If the C function does not return any value in the current program, then the default void () function is used.
function_name – The name of the function is user defined, which the C programmer declares as per his requirement. However, the function name is a valid C identifier at the time of argument calling in any C program.
parameter_list – The function parameter list in C is the list of program variable argument parameters (arguments) accepted by the function. Each C program parameter has different types of data types and variable parameter names. Which are separated by the comma operator.
Example.
// Function declaration type
int total(int p, int q);
void hello(char emp_name[]);
In above example.
total – The total variable declare is a function that returns an int value and takes two int arguments/parameters (p and q).
hello – The hello declare is a function that returns void (no program return value) and takes a single parameter, a char emp_name array (emp_name[]).
Function Definition in C.
Function definition provides the actual execution of the program function. It includes the main portion of the function declaration in C program. Which contains function statements that define what the function will do when called in the current program.
Function definition syntax.
return_type function_name(parameter_list) {
// function body (function_statement)
}
return_type – This defines the data type of the value to be returned by the declared function. If the function does not return any value to the program, then the default void () function is used.
function_name – The name of the function declared in the program, matching the function declaration.
parameter_list – List of function parameters with their data types and function variable names, matching the function declaration.
Function definition example.
// Function definition for the total function
int total(int p, int q) {
int add = p + q;
return add;
}
// Function definition for the count function
void count(char emp_name[]) {
printf(“\n count, %s!”, emp_name);
}
Calling the function.
In function calling declare function While using a function in the program, you simply call it by its name. If required in the program declare function parameter argument value is passed. Otherwise you call the function without passing function argument.
Function calling example.
int main() {
int count = total(1, 2); // Calling the total function
printf(“\n count of total – %d\n”, count);
hello(“welcome”); // Calling the hello function
return 0;
}
Some important points of function calling.
function prototype – In C program function prototype has to be declared before its first use if the function definition appears later in the program code or in a separate file. It indicates to the C compiler about the digital signature of the function.
// c Function prototype example
int total(int p, int q);
void hello(char stu_name[]);
Recursive Function in C – Recursive functions can be used in C programs where a recursive function declared in a C program can call itself directly or indirectly through other functions.
function parameter – Variable arguments passed to a function in a program are generally passed by program value, which means that changes to parameters declared within a function in a program do not affect the original arguments, unless function pointers are used in the program.
Function overloading – Unlike some other popular programming languages, C does not support function overloading, where multiple functions in a single program can have the same name but different argument parameter lists.
Function return values - Functions can return values of any data type, including primitive data types (int, double, char, etc.) and user-defined data types (structures, pointers), etc.