Logical operators
Logical operators in C programming are used to perform logical operations on the boolean values (true or false) of variables used in the program. Logical operators are generally used to merge or combine multiple programming conditions together or to negate conditions.
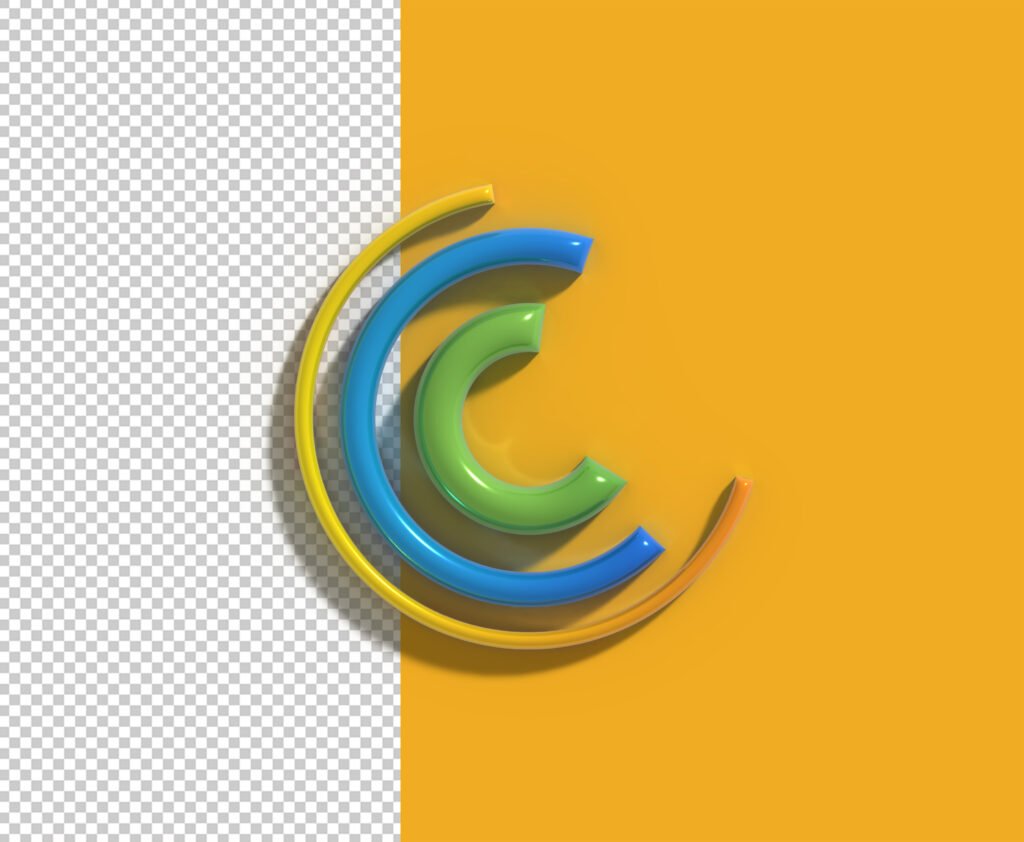
Types of Logical Operators in C Programming.
Logical AND (&&) Operator.
A logical AND operation is used in a C program condition. If both program variable operands are true at the same time, then it returns a true program output value; otherwise, it returns a false program output value.
Logical AND (&&) Operator Example in C Language.
int p = 3, q = 7;
if (p > 1 && q > 1)
{
// Both program if conditions are true
}
Logical OR (||) Operator in C.
A logical OR in C language performs a program condition operation. If the given program condition and at least one of the or condition operands are true, it returns the true value as output; otherwise, it returns the output as false.
Logical OR (||) Operator Example in C Language.
int p = 1, q = 4;
if (p > 1 || q >1 )
{
// At least one of the or program conditions is true
}
Logical NOT (!) Operator in C Language.
A logical NOT operation in C program performs a logical condition. It reverses the logical condition of its program condition operands. If the given program NOT operand is true, it returns a false value output, and vice versa.
Example of logical NOT (!) operator in C.
int p = 1;
if (!(p > 5))
{
// here The given condition is true because !(p > 5) is true
About Logical Operators in C.
Logical operators are often used in C language to merge or exclude program conditions in conditional statements (if, while,for) loops.
Whereas the logical AND (&&) and logical OR (||) operators in C are like a short-circuit, which means they stop evolving the program condition as soon as the output is known.
The logical NOT (!) in C is a unary program operator, which means it only operates on one program operand at a time.
Most logical operators are typically used with Boolean expressions in a program, but they can also be used with integer expressions (0 is considered false, 1 is considered true).