Conditional compilation in c hindi
C में कुछ विशेष प्रोग्राम कंडीशन में कंडीशनल संकलन आपको कुछ कंडीशंस के आधार पर मैक्रोज़ प्रोग्राम कोड के कुछ हिस्सों को प्रोग्राम में ऐड या रिमूव फीचर्स प्रदान करता है। यह मैक्रोज़ प्रोग्राम कंप्यूटर प्लेटफ़ॉर्म-विशिष्ट कोड, प्रोग्राम डिबगिंग और कोड को कस्टमाइज करने के लिए विशेष रूप से उपयोगी है। यहाँ आपको कुछ कंडीशनल संकलन के लिए उपयोग किए जाने वाले प्रीप्रोसेसर डायरेक्टिव दिए गए हैं.
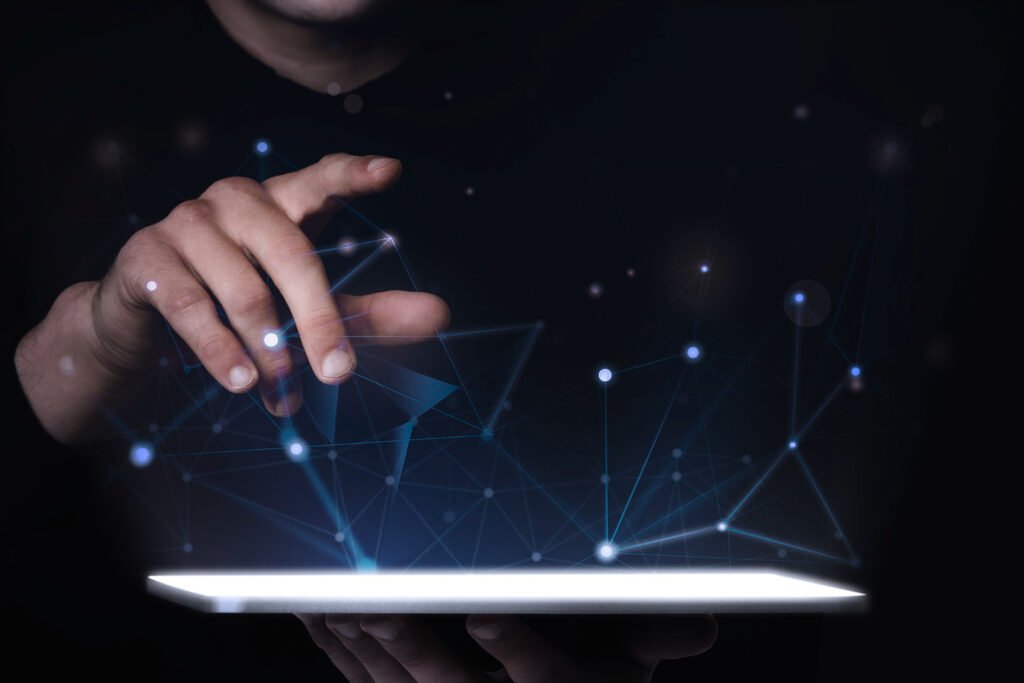
These are some popular conditional preprocessor directives.
#ifdef and #ifndef
#define and #undef
#if, #elif, #else, and #endif
Basic conditional compilation in macros.
#ifdef और #ifndef प्रीप्रोसेसर डायरेक्टिव का उपयोग करना
#ifdef प्रीप्रोसेसर डायरेक्टिव यह जाँचता है, कि क्या मैक्रो डिफाइन है।
#ifndef प्रीप्रोसेसर डायरेक्टिव जाँचता है, कि क्या मैक्रो डिफाइन नहीं है।
#include <stdio.h>
#define DEBUG
int main() {
int p = 4, q = 7;
#ifdef DEBUG
printf(“\n The Debug is – p = %d, q = %d\n”, p, q);
#endif
int total = p + q;
printf(“\n the total is – %d\n”, total);
return 0;
}
यहाँ इस उदाहरण में, डीबग मैसेज केवल तभी प्रिंट होता है, जब DEBUG को डिफाइन किया गया हो।
सी मैक्रोज़ में #if, #elif, #else, और #endif प्रीप्रोसेसर डायरेक्टिव का उपयोग करना
#if प्रीप्रोसेसर डायरेक्टिव जाँचता है कि कोई कंडीशन ट्रू है या फाल्स है।
#elif प्रीप्रोसेसर डायरेक्टिव सेकंड कंडीशन टेस्ट है यदि पिछली #if या #elif शर्त फाल्स थी।
#else यह आपको एक विकल्प प्रदान करता है, यदि पिछली कोई भी प्रोग्राम कंडीशन ट्रू नहीं थी।
#endif यह कंडीशनल ब्लॉक के अंत को चिह्नित करता है।
#include <stdio.h>
#define TEST 3
int main() {
#if TEST == 1
printf(“\n TEST 1\n”);
#elif TEST == 2
printf(“\n Test 2\n”);
#elif TEST == 3
printf(“\n Test 3\n”);
#else
printf(“Test other\n”);
#endif
return 0;
}
ऊपर दिए गए उदाहरण में आउटपुट टेस्ट के मान पर प्रोग्राम निर्भर करता है।
Macros Platform-specific code example.
सी मैक्रोज़ प्रोग्राम में कंडीशनल संकलन का उपयोग अक्सर प्लेटफ़ॉर्म-विशिष्ट प्रोग्राम कोड को ऐड करने में किया जाता है।
#include <stdio.h>
int main() {
#ifdef C
printf(“\n test on c Windows”);
#elif JAVA
printf(“\n text on java”);
#elif PYTHON
printf(“\n test on python”);
#else
printf(“\n no above platform supportd”);
#endif
return 0;
}
C macros debugging example.
आप सी प्रोग्राम में डिबगिंग प्रोग्राम कोड को ऐड करने के लिए कंडीशनल प्रोग्राम संकलन का उपयोग कर सकते हैं. जो रिलीज़ संस्करण में मौजूद नहीं होना चाहिए।
#include <stdio.h>
void debugLog(const char *msg) {
#ifdef DEBUG
printf(” the DEBUG – %s\n”, msg);
#endif
}
int main() {
debugLog(“begain the program”);
int p = 4, q = 3;
int total = p + q;
debugLog(“the total count is”);
printf(“\n the total is – %d\n”, total);
debugLog(“let terminate the program”);
return 0;
}
C macros debugging example.
सी मैक्रोज़ प्रोग्राम अनुकूलन के लिए कोड को ऐड या एक्सक्लूड करने के लिए कंडीशनल संकलन का भी उपयोग कर सकते है।
#include <stdio.h>
#define Let_OPTIMIZE
int main() {
#if LET_OPTIMIZE
printf(“\n This is Optimized code\n”);
// use Optimized algorithm
#else
printf(“\n This is the Non-optimized code”);
// Simple optimize algorithm declaration
#endif
return 0;
}
सी मैक्रोज़ में #define और #undef का उपयोग।
#define एक यूजर डिफाइन मैक्रो बनाता है।
#undef मैक्रो डेफिनिशन को हटाता है।
#include <stdio.h>
#define DEBUG
int main() {
#ifdef DEBUG
printf(“\n program Debug mode is on”);
#endif
#undef DEBUG
#ifdef DEBUG
printf(“\n This statement no printed”);
#endif
return 0;
}
C Macros Include Guard Example.
यदि आप किसी मैक्रोज़ में एक से अधिक गार्ड एक ही हेडर फ़ाइल के कई समावेशन को रोकना चाहते हैं। तब इसका इस्तेमाल करे.
#ifndef HEADER_FILE_NAME_H
#define HEADER_FILE_NAME_H
// Macros header file content
#endif // HEADER_FILE_NAME_H