what and how to java language
How to declare array in java
Declaring an array in a Java program requires you to provide the declared array variable name, the number of declared or held-on array storage values, and the data type of the defined array element in your Java program.
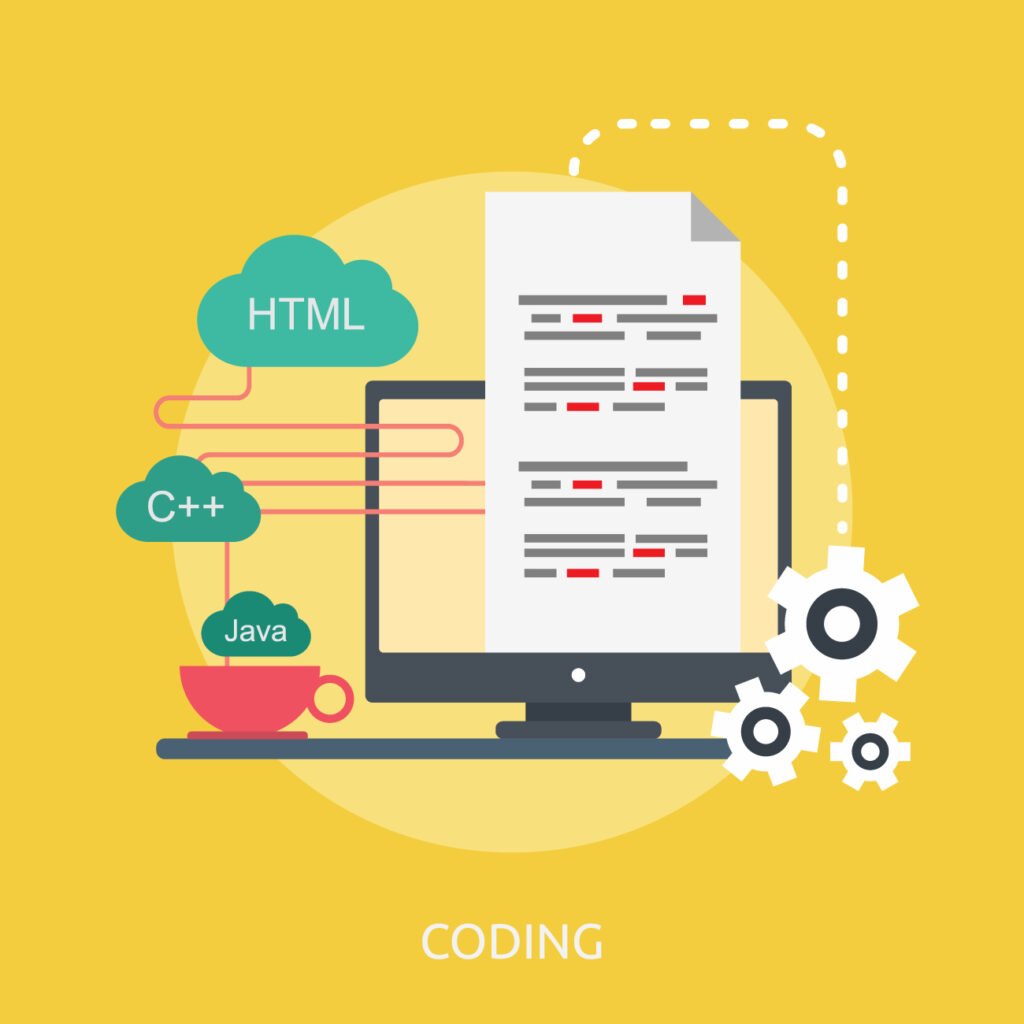
Below is the syntax for declaring a straightforward array in a Java application.
Syntax –
aray dataType[] arrayName;
// simple integer array declaration in java
int[] simplearray;
or
int testarray[];
//simple string array declare in java.
String datatype [] variable name;
or
String course[];
// simple array declare in java language.
int[] data = {23, 45, 67, 49, 95, 88};
and
int[] data; // simple array declaration
data = new int[]{0, 5, 7, 9, 25, 78}; // java array initialization
What is inheritance in java
A basic idea in object-oriented programming (OOP) languages like Java is inheritance. It enables one class to inherit the characteristics (methods and fields) of another class that has been declared in a Java program. This is referred to as the parent class or superclass. A subclass or child class is a class that derives from a superclass.
The extends keyword is used to implement inheritance in Java. The fact that a subclass may access and utilize both the members (methods and fields) of the superclass and those of its own class indicates that it extends the superclass.
The Java Language syntax for defining a subclass descended from a superclass is provided below.
superclass.
class Subclass extends Superclass //let’s declare a super class
{
// add here member of subclass members
}
The functionality of the superclass may then be expanded and specialized by the subclass by adding new methods and fields.
Inheritance encourages a class hierarchy and enables for code reuse. As a result, you may build more specialized and particular classes out of more basic classes, helping to organize the code and cut down on repetition.
Consider a superclass with the name Course that has common properties and methods associated with the name of the superclass. Superclasses like Software Course, Web Development Course, and Database Management may have subclasses that can be created within them. the courses that may be found in Superclass. Each subclass may have additional traits or characteristics unique to that particular course type.
Polymorphism, which involves using an object wherever the superclass object is expected to be used, is another benefit of inheritance. A subclass object is usable. You may develop computer code that is more scalable and modular as a result.
What is static in java, what is a static method in java
The static keyword is used to declare certain members (variables and methods) in Java Language. which refer to the class itself as opposed to class instances. These static members are also referred to as class members on occasion.
Basic Java Programming Uses and Purposes for Static Keywords.
Static variable (Class variable).
- All instances of a class share static variables.
- They are only initialized once, upon the loading of the class, and are declared using the static keyword.
- They may be accessed immediately by using the class name, without having to create an instance of the class.
- Constants, counters, and shared data are examples of static variables that are often used.
Static Methods (Class Methods).
- Static methods are inherent to the class and do not belong to any particular instance.
- Without establishing a class instance, they are defined using the static keyword and may be invoked directly using the class name.
- Static methods are often used for utility methods, auxiliary functions, or operations.
- they can only directly access other static members (variables or methods) of the class. which don’t need access to data that is exclusive to a certain instance.
What is encapsulation in java
Java and other object-oriented computer programming languages are built on the idea of encapsulation. In Java and other object-oriented computer programming, encapsulation is used to gather together program data (variables) and program procedures (program methods) while restricting access to them from outside the class. The Java language encourages the idea of data variable hiding and abstraction through encapsulation.
How to initialize an array in java
When declaring an array data type variable or later, Java programmers can initialize it using a variety of techniques. Here are a few Java application initialization methods for arrays.
At the moment the program is declared, initialize the array.
Curly brackets can be used to directly indicate the array values when declaring an array in a Java application.
The size of an array in this Java application is automatically calculated based on the number of specified array variable members.
dataType[] declarearrayname = {arrayvalue1, arrayvalue2, arrayvalue3, arrayvalue4,…};
Let’s declare and initialize a simple java array.
int[] arrayvalue = {2, 9, 0, 7, 5};
Initialize array with the new keyword.
When initializing an array in a Java application, you must do it before declaring the array. Therefore, you may allocate memory and give an array element a value using the new keyword.
There must be a specification of the array’s size.
dataType[] declarearrayname = new dataType[size];
Basic array example.
int[] arraynum = new int[7]; // let’s declare Array of size 7 integer elements
numbers[0] = 22;
numbers[1] = 44;
numbers[2] = 56;
numbers[3] = 77;
numbers[4] = 99;
numbers[5] = 75;
numbers[6] = 87;
How to throw exception in java
In a Java application, you may explicitly throw an exception by using the throw keyword. You can raise a Java Program Exception by doing this. when a specific program condition or logic is present in your program code.
An illustration of how to throw an exception in a Java application is shown below.
A Java software exception is an example.
public void testprice(float price) throws IllegalArgumentException {
if (price < 0) {
let’s you can throw new IllegalArgumentException(“price is not negative”);
}
// apply some other program logic here
}
The testPrice method in the earlier mentioned software sample determines whether or not the supplied price is negative. In such case, the current Java software issues an IllegalArgumentException with a warning that the testprice cannot be in the negative order.
How to throw an exception in java
In Java, you may use the throws keyword followed by an instance of the specified exception class to throw a program exception.
A Java application sample showing how to throw an exception is provided below.
The suitable exception class is chosen here.
The built-in exception classes offered by Java Language allow Java developers to manage a wide range of unusual programming logic and situations. By extending the Exception class or one of its subclasses, you may also make your own exception class.
Select the exception class that most accurately reflects the exceptional circumstance your Java application should handle.
To throw an exception in a Java application, use the throw keyword.
Use the new keyword to create an object of the chosen exception class.
Use the throw keyword and the exception object to throw an exception.
An example of throwing an exception in fundamental Java is provided here.
public void dividenumber(float dividend, float divisor) throws and ArithmeticException java program exception
{
if (divisor == 0) {
throw new ArithmeticException(“division with zero not permitted”);
}
int output = dividend / divisor;
System.out.println(“the outpute is – ” + output);
}
The divideNumber method in the example above determines whether or not the divisor is zero. If this is the case, a Java program that already exists throws an ArithmeticException with a particular error message stating that division by zero is not permitted.
A throws clause in the method signature may be used to propagate the exception to the caller code or to catch and handle exceptions when they are thrown using a try-catch block. if the exception is not recognized or dealt with. As a result, it will continue to move up the call stack until it is stopped or the program ends.
What does ++ mean in java, what is ++ in java
The ++ operator is sometimes referred to as the increment operator in Java language. One increment is added to a program variable’s value using the increment operator. Both character and numeric variables that are defined in Java applications can be used with the ++ operator.
In Java, there are two types of increment operators.
Pre-increment(++variable).
- The pre-increment Java operator raises a declared program variable’s value by 1 before doing any additional operations.
- The expression is then modified by the higher value.
Pre-increment example.
int p = 1;
int output = ++1;
System.out.println(output);
// Output is – 2
Post-increment(variable++).
- Any Java application uses the declared program variable’s value in the expression before incrementing it by the specified increment value.
- The variable’s initial value is utilized in the expression before it is increased.
Java program example of a post increment.
int q = 2;
int output = q++;
System.out.println(output);
// Output is – 3
System.out.println(q);
// Output is – 2
What is overriding in java
In Java Language, one such feature is method overriding. This enables a Java subclass to offer a unique implementation of that method. which its superclass has previously defined. when a method in a subclass has the same name, return type, and list of program variable inputs as a method in a superclass. Overriding the superclass method is what it is known as.
The following are some essential ideas concerning Java method overriding that you should comprehend.
- Inheritance – Java’s idea of method overriding and inheritance are closely connected. when a subclass is created that inherits the attributes and functions of its superclass.
- Signature – When overriding a method, the subclass version must have the same method name, return type, and argument list as the superclass version.
- Access modifier – The subclass method that is overridden must have the same access as or more access than the superclass method. For instance, if a method from a superclass is made public. Therefore, a subclass’ overriding method can be specified as public or protected but not private.
- @Override annotation – When overriding a method, @Override annotation is advised to be used. This annotation aids in verifying that a method from the superclass is indeed being overridden and assists in identifying issues when the method signatures do not match.
- Method invocation – When a method that has been overridden is used. Therefore, the subclass version of the method is used instead of the superclass version. Runtime polymorphism or dynamic polymorphism refers to this type of thing.
Below is an example that demonstrates method overriding in Java.
class Course {
public void selectcourse() {
System.out.println(“You can selectcourse”);
}
}
class Java extends course {
@Override
public void selectcourse() {
System.out.println(“You can select java”);
}
}
public class Main {
public static void main(String[] args) {
Course course = new Course();
course.selectcourse(); // Output: Animal makes a sound
Java java = new Java();
java.selectcourse();
Course anotherCourse = new Java();
anotherCourse.selectcourse();
}
}
The Java class in the previously mentioned Java application example extends the Course class and replaces the selectCourse() function. when a Java object’s selectCourse() function is used. Therefore, the Java class executes the override version. Additionally, when a course reference points to a Java object calls the selectCourse() function. In order to accomplish polymorphism, the overridden version of the Java class continues to run.
What does // mean in java
Java applications utilize the double forward slash (//) to denote a single-line program remark. The Java language has this functionality. It enables Java programmers to include illustrative comments for program statements within their program code.
When the // character appears in the source code, the Java compiler. The remainder of that line is therefore treated as a remark and ignored when the program is being executed. These comments are used by programmers and Java developers to better understand the program code; they are not actually executed as part of the Java program.
Here you will find an example of a single-line comment in Java.
public class addition {
public static void main(String[] args) {
int p = 7;
int q = 3;
// let’s calculate the sum of p and q integer variable
int add = p + q;
System.out.println(“The addition is – ” + add); // it print the result add p and q variable
}
}
Comment // Calculate the sum of the p and q variables in the program code above. A quick explanation of what it performs is given in the line of code that follows. A similar explanation of the println statement can be found in the remark //Print the result.
For big Java program code documentation, comments are required. hence improving the readability, comprehension, and maintainability of existing Java program code for both the original developer and additional users who will deal with the code in the future.
What is a java bean
A Java application class is called JavaBean. It follows to a set of standards established in the Java Beans specification. It is a design pattern and coding convention for Java that allows the creation of modular programs or reusable program components.
Here, in the section below, is a simple JavaBean class example.
public class Person implements java.io.Serializable {
private String name;
private int age;
public Person() {
// Default constructor
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
The Employee class in the aforementioned Java application example is a JavaBean. Name and age are examples of private fields with matching getter and setter methods. Additionally, it uses the Java.io API. interface that is serializable to facilitate serialization.
What is thread in java, what is a thread in java
A distinct flow of execution within a program is referred to as a “thread” in Java Language. It enables Java programmers to run many tasks or sections of a Java application concurrently or in parallel. Java programmers may generate and manage many threads thanks to Java’s threading technology. This enables responsiveness in applications, effective resource usage, and concurrent execution of Java computer code.
Java threads are instances of the java.lang package is a subclass of the thread class. A separate path of execution within a program is represented by a thread. It has the ability to run code simultaneously with other threads. Each thread has its own local variables, stack, and program counter.
Below you are given a simple example, which shows creating and starting a thread in a Java program.
public class TestThread extends Thread {
public void run() {
System.out.println(“The created Thread is running”);
}
public static void main(String[] args) {
TestThread thread = new TestThread();
thread.start(); // Start the thread
}
}
The TestThread class extends the Thread class in the preceding Java application example and overrides the run() function. The program that will be run on the new thread is contained in the run() method. When a thread is started, the start() function is used, and it also calls the run() method of the main thread.
What is an expression in java
In Java Language, an expression is made up of a literal, a variable, an operator, and a method call. It generates value. It is an enumeration or computation. This may be assessed to yield outcomes.
The phrase can be applied in several situations. There are several additional operations available, including assignment, method logic, conditional statements, and loop conditions.
Below you are given some examples of basic expressions in Java programs.
Java Arithmetic Expressions Example.
int add = 1 + 2;
long double result = 7.50 * (1.0 / 2.0);
How to declare an array in java
Declaring an array in a Java application involves identifying the kind of entries it will contain. The name of the array variable can then be specified, followed by square brackets ([]). Depending on the requirements, there are a number alternative ways to declare an array.
You will find several examples below.
Declaring Java Array Size and Initialization.
int[] numeric = new int[7]; // here we declare an integer array with a size of 7 element
String[] emp_names = new String[30]; // here we declare a string array name with emp_names a size of 30 elements
The array number and array name are specified with a specific size in the previous example. A new array object of the requested size is created using the new keyword.
Declaration with array initialization.
int[] numeric = {99, 33, 56, 23, 98, 89}; // hare seclare and initialize an integer array with elements
String[] stu_names = {“John”, “Robert”, “David”, “Mathew”}; // here we declare and initialize a string array with student name
Here, specified values encased in curly brackets are used to declare and initialize the array’s names and numbers. The number of items supplied at startup determines the array’s size.
What is this in java
Java Language keywords It serves as a pointer to the active object instance. It may be used to refer to that object in a constructor or instance method. which the constructor or function is being called.
What is java used for
Java language is a flexible, all-purpose. It has several applications in programming.
Below are a few typical applications for Java programming.
- The creation of applications.
- Web development and business software.
- The development of Android.
- Processing of Big Data.
- IoT, or the Internet of Things.
- Computing using numbers and science.
- Game creation.
- Financial Software.
- Instruction.
What is serialization in java
In Java programming, the process of turning an object into a byte stream is referred to as serialization. which may be quickly saved to a file, transferred across a network, or saved to a computer using a persistent storage device. This preserves the object’s state and behavior so that it may be rebuilt later, possibly in a different Java Virtual Machine (JVM).
The class that has to be serialized must implement the java.io.Serializable interface in order to complete the serialization process. The class’s serializability is denoted by this interface, which serves as a marker interface.
What is abstraction in java
An essential idea of object-oriented programming is abstraction in Java programming. It enables the simplification of complicated programming systems’ representation. It focuses on capturing the key characteristics and actions of a system or object while obscuring extraneous information.
In Java programming, abstraction is accomplished via two primary approaches. Java uses abstract classes and interfaces.
What are instance variables in java, what is an instance variable in java
In Java language, instance variables are often referred to as member variables or instance fields. variables declared outside of a method or constructor but inside of a class. These include information particular to each object (instance) of the class. The instance variables of a class are duplicated for each instance, independent of other instances.
What is garbage collection in java
In Java language, garbage collection is a system for automatically managing memory. In order to avoid having to actively deallocate program memory and control object lifecycles, Java programmers may now do so. It automatically recognizes and obtains the memory from the Java application. which the software object no longer uses, enabling the system to effectively utilize the memory resources available.
What is package in java, what is a java package, what is a package in java
A package is a mechanism to group similar classes and interfaces into a single namespace in Java programming. It gives programmers a way to organize similar code into groups and prevents naming conflicts between classes with the same name.
How to sort arraylist in java
Java programmers can use the Collections.sort() method of the java.util.Collections class to sort the items of an ArrayList. The ArrayList’s elements are sorted using the Collections.sort() function in ascending order, either using the elements’ own natural order or a custom Comparator.
Here is a Java example of how to rank numbers in an array list in ascending order.
import java.util.ArrayList;
import java.util.Collections;
public class ArrayListSortExample {
public static void main(String[] args) {
ArrayList<Integer> integer = new ArrayList<>();
integer.add(10);
integer.add(9);
integer.add(5);
integer.add(7);
integer.add(0);
integer.add(1);
integer.add(2);
System.out.println(“Random number order – ” + integer);
Collections.sort(integer);
System.out.println(“After sorting all integer element – ” + integer);
}
}
What are the primitive data types in java
In Java language, there are eight primitive data types. one of the numerous data types in Java is the most fundamental. They do not represent program objects or class instances, instead, they represent simple programming values.
The following are the Java data primitives.
- Boolean – Represents a Boolean data type’s value. In Java, it denotes either a true or false condition.
- Byte – In Java, a byte variable denotes a signed 8-bit integer value. Its range is between -127 and -128.
- Short — In Java, the short keyword denotes a signed 16-bit integer value. It falls between -32,768 and 32,767.
- int – In Java language, int stands for a signed 32-bit integer value. Between -2,147,483,648 and 2,147,483,647 is its range.
- long – In Java, long denotes a 64-bit unsigned integer value. -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 are the possible values.
- float – In Java, the float keyword denotes a 32-bit floating-point value with single precision. It is helpful for a moderately accurate representation of decimal numbers.
- double – A double-precision 64-bit floating-point value is represented by this type in Java. It helps with a very accurate representation of decimal numbers.
- Char – In Java, this class represents a single character from the Unicode character set. It keeps values for 16-bit Unicode.
Below you are given an example, which demonstrates the use of primitive data types in Java.
public class PrimitiveTypesExample {
public static void main(String[] args) {
boolean flag = true;
byte a = 127;
short srt = 32767;
int integer = 2147483647;
long longinteger = 922337203686676574L;
float floating = 3.14f;
double doublevariable = 3.14159;
char charvariable = ‘V’;
System.out.println(“output of primitive data type in java\n”);
System.out.println(“boolean data type – ” + flag);
System.out.println(“byte data type – ” + a);
System.out.println(“short data type – ” + srt);
System.out.println(“int data type – ” + integer);
System.out.println(“long data type – ” + longinteger);
System.out.println(“float data type – ” + floating);
System.out.println(“double data type – ” + doublevariable);
System.out.println(“char data type – ” + charvariable);
}
}
How to install java on ubuntu
If you wish to use the Ubuntu Linux operating system with Java applications. Therefore, you can follow the instructions below.
Update the package index.
sudo apt update
Install your Linux default Java Development Kit (JDK) package.
sudo apt install default-jdk
This command installs the OpenJDK package, which is the default implementation of Java in Ubuntu.
Verify Java program installation.
java -version
This command will display the Java version installed on your Ubuntu Linux.
In addition, you may launch Java applications without the requirement for development tools. The Java Runtime Environment (JRE) package can then be installed.
sudo apt install default-jre
Depending on your requirements, you can also decide to install a certain Java version or utilize an alternative Java implementation, such as the Oracle JDK or OpenJDK.
What is a constant in java
A variable with a constant data type in Java language has an assigned value that cannot be modified. In Java applications, it stands in for a fixed value. This doesn’t change while a program is running. Usually, the values are kept as constant variables. Those that aren’t anticipated to change, such setup options, established values, or mathematical constants.
In Java applications, constants are specified using the final keyword. This implies that it is impossible to change the program variable’s value. To impose immutability, the final keyword can be used on variables, methods, and classes.
Below you are given an example of declaring a constant variable in Java programming.
public class ConstantsExample {
public static final int Int_VALUE = 2456;
public static final double PI_value = 3.14159;
public static final String text = “Welcome to vcanhelpsu.com”;
public static void main(String[] args) {
System.out.println(“Integer value – ” + Int_VALUE);
System.out.println(“PI value – ” + PI_value);
System.out.println(“Text value – ” + text);
}
}
The final keyword is used to define the variables Int_VALUE, PI, and Text as constant variables in the Java program example above. Once a value is assigned to these variables. Thus, the software cannot alter their values.
How to call a method in java
If you wish to use a Java program’s methods. The primary requirements are hence these steps.
Make a new instance of the class that contains the method, or, if one already exists, use it. If the method is static, the class name can be used to invoke it directly.
To access a method from an instance or class, use the dot operator (.).
Include any necessary arguments in parenthesis and pass them to the method. You can omit the parenthesis if the method doesn’t require any parameters.
whether the technique yields a result. As a result, you may utilize it directly or save it in a variable.
Below you are given a program example of how to call a method in Java.
public class MyClass {
public void testMethod(String info) {
System.out.println(“Text info is = ” + info);
}
public static void main(String[] args) {
MyClass obj = new MyClass(); // Step 1 let Create an instance of the class
obj.testMethod(“Welcome to the vcanhelpsu.com”); // Step 2 and 3 now Call the method and pass a parameter
}
}
The TestMethod is called on the obj instance of the MyClass class in the previously mentioned software example. The phrase “Welcome to the vcanhelpsu.com!” is sent as a parameter. The message is then printed to the console using the technique.
What is parameter in java, what is a parameter in java, what are parameters in java
A declared variable value is a parameter in the Java language. Which is supplied when a method is called. By doing this, you may give the method input that it can utilize to carry out any necessary operations or computations. By enabling you to work with various program data values, parameters help you to make your procedures more flexible and modular, allowing you to reuse program code.
The method’s signature has a list of parameters enclosed in parenthesis and separated by commas. The name of each argument is followed by the Java program data type. The value type is specified by the data type. what that parameter’s method requirements are.
Below you are given an example of a method with parameters in a Java program.
public void printMessage(String message, int count) {
for (int i = 0; i < count; i++) {
System.out.println(message);
}
}
The method TextMessage in the Java application example above contains two arguments. Declare program variables count and message with the data types int and string, respectively. Both arguments must be filled out when calling this function.
printMessage(“Welcome to vcanhelpsu.com”, 5);
In the program software example, the count argument is set to 5, therefore the method will print the message “welcome to vcanhelpsu.com” five times.
What is a float in java
Java programming uses the primitive data type float. In any Java application, which represents single-precision floating-point integers for defined float data variables. It is used to hold decimal integers with fractional parts when float variables are present. In comparison to the Int and Long data types, the Float data type has a larger range and more precision. It is a 32-bit number.
Java program variables of the float data type are specified with the keyword float.
Here is an example of declaring and initializing a float variable:
float sampleFloat = 10.19f;
The float variable sampleFloat is used in the program example. It has the variable value 10.19 initialized. Keep in mind that the suffix f must be used to denote the float type of the literal value. Java considers the value as a double, a bigger data type, without the F suffix.
Below is an example of using float variables in arithmetic operations.
float p = 6.10f;
float q = 3.7f;
float add = p + q;
float sub = p – q;
float mul = p * q;
float div = p / q;
System.out.println(“the Addtion is – ” + add);
System.out.println(“the subtraction is – ” + sub);
System.out.println(“the multiply is – ” + mul);
System.out.println(“the division is – ” + div);
What is casting in java
In Java language, casting describes the procedure of transforming a value from one data type to another. By using this, you may pretend that a program value is a different data type for the time being. Java offers two different kinds of casting support. Casting might be broadening (implicit) or narrowing (explicit).
Widening (Implicit) Casting.
- This occurs when a value is changed in a Java application from a smaller to a larger data type.
- The Java compiler runs it automatically. because the amount may be raised without compromising accuracy or data.
- For instance, a float may be changed into a double and an int could become a long.
Narrowing (Explicit) Casting,
- Occurs when you change a value in a Java application from a bigger data type to a smaller data type.
- Because doing so might lead to accuracy or data loss, you must explicitly state that you are lowering the value of the program data variable.
- Converting a double to an int or a long to a short, for instance.
- The (targetType) value syntax is used for explicit casting. One illustration is (int) testDouble.
Below you will find examples that demonstrate casting in Java.
int myInt = 100;
long myLong = myInt; // Widening casting (implicit)
double myDouble = 3.14;
int myInt2 = (int) myDouble; // Narrowing casting (explicit)
float myFloat = 5.67f;
int myInt3 = (int) myFloat; // Narrowing casting (explicit)
The int value 500 in the previously mentioned software example is automatically converted to a long and allocated to the testLong variable. There is no need for explicit casting because this is an illustration of widening (implicit) casting.
What is variable in java, what is a variable in java
A named storage location stated in a Java program is referred to as a variable in that language. It keeps a value of a specific data type. A Java program variable serves as a container to hold and alter the contents of a defined variable’s data while a program is being executed. Java applications can store and retrieve data using variables. which the application might utilize for computations, control structures, and other tasks.
You must first define a variable with a data type before utilizing it in a Java application. where the variable’s name and data type must be supplied. Java is a statically typed programming language, hence every variable that is declared in Java must have its type specified explicitly.
Here you will find below the syntax to declare a variable in Java programming.
Data Type Variable Name;
For example in Java, to declare an integer variable named p, you would write.
int p;
In the above example, int is the variable data type, and p is the variable name. The int data type represents integer (integer) data storage in Java.
After declaring a variable in Java, you can assign a value to it using the assignment operator (=).
Here’s an example.
p = 1;
In this case, the value 1 is assigned to the p variable.
Alternatively, you can declare and initialize a variable on one line.
int q = 2;
You can declare variables of reference kinds, such as object, array, and custom classes, in addition to the Java primitive data types (such as int, double, boolean, etc.).
In a Java program, program variables can be applied in a number of different ways. Input from the user is stored, computations are done, interim findings are kept, and more. They can also be given new values while a Java application is running.
It is important to remember that each declared variable in a Java program has a scope. This describes how accessible and visible they are within the software. Where a variable may be used and accessed depends on its scope.
What is maven java
For Java applications, Maven is a well-liked build automation and dependency management solution. It offers a standardized method for managing the build process, dependencies, and project setup for Java programs. The Maven software facilitates the development process by automating processes including source code compilation, application packaging, and management of external libraries.
How to initialize a list in java
Depending on your individual requirements and the Java version you are running, there are various possible ways to begin a List data type program in a Java program. Here are a few typical Java methods for initializing lists.
Initializing a List with ArrayList.
Using the ArrayList class from the java.util package, you can create an empty list, or initialize it with some initial array elements.
Here you are given an example below.
import java.util.ArrayList;
import java.util.List;
// here we declare empty list
List<String> emptyList = new ArrayList<>();
// declare list with initial array elements
List<Integer> numerics = new ArrayList<>(List.of(11, 12, 13, 14, 15, 16, 1 7));
In the example, the numbers are initialized as Integer 11, 12, 13, and 14 using the constructor accepting a collection, and the Empty List is initialized as an Empty ArrayList using the default constructor. began by using arraylists 16 and 17.
What is n in java
In Java programming, n is not a predefined keyword or variable. This is only a convention that is frequently used as a variable selection name for Java programs. to indicate a number or count, particularly when looping or mathematical ideas are involved. Any legal variable name that complies with Java’s naming standards may be used in place of the arbitrary variable name n. Along with correct variable declaration, Java application also accepts it.
Here you will find an example of using n as a loop counter variable with a Simple for Loop.
for (int n = 0; n <= 9 ; n++)
{
System.out.println(n);
}
The int variable n is utilized as a loop counter in the program example sample. From iteration 0 to iteration 9, the loop will report the value of n to the output.
What is method overloading in java
Java applications’ ability to declare numerous methods with the same name but differing program variable argument lists is known as method overloading. This enables you to offer several method implementations depending on the kind, quantity, or arrangement of variable parameters.
When a program method is overloaded, other methods with the same name but different signatures are created. A method’s name and the types of its parameters make up its signature. When considering method overloading, the return type of a method is not taken into account.
Below you are given an example in Java, which demonstrates method overloading.
public class summation {
public int add(int p, int q) {
return p + q;
}
public double add(double x, double y) {
return x + y;
}
public int sum(int l, int m, int n) {
return l + m + n;
}
}
The summation class defines three add methods in the Java application example above. However, the list of parameter variables differs for every method. The first method, add(int p, int q), accepts two integer values and outputs their total as an integer. Taking two double variable values and returning a double amount is the second method, add(double x, double y). Taking three integer values and returning an integer sum is the third way, sum(int l, int m, int n).
Java chooses an overloaded method to use when you call the add method based on the parameters you supply. Based on the type, quantity, and sequence of the inputs, the compiler matches them to the relevant method.
What is /n in java
A newline character is represented in an existing Java program via the escape sequence “n” in Java Language. When publishing output to the console or a file, it is used to break up lines in Java programs and to advance the cursor to the following line.
when a string contains the character n. It so serves as a unique character. It denotes a newline or line feed.
For example, consider the following Java program code.
System.out.println(“Welcome to vcanhelpsu.com”);
The output of this code will be:
welcome
to
vcanhelpsu.com
Java programs often use \n escape sequences to format text or add new lines. To produce a more complicated output, it can be concatenated with other characters and strings.
What is biginteger in java
BigInteger is a built-in class in the java.math package in Java Language. which supports numbers with any degree of precision. This gives you the flexibility to deal with integers of any size, with the sole memory restriction being their size. BigInteger can handle integers of any size, unlike primitive integer types like int and long, which have restricted ranges. On big numbers, it offers operations for arithmetic, comparison, and other mathematical processes.
Below is an example of how to use BigInteger in Java Language.
import java.math.BigInteger;
public class BigIntegertest {
public static void main(String[] args) {
BigInteger p = new BigInteger(“798734213531231812381”);
BigInteger q = new BigInteger(“472354865347648622436”);
// let’s do some addtion operation with big integer variable
BigInteger sum = p.add(q);
System.out.println(“The big integer add is – ” + p);
// let’s multiply some big integer variable
BigInteger product = p.multiply(q);
System.out.println(“The multiply of big integer – ” + product);
}
}
We construct two BigInteger objects, p and q variables, with BigInteger values in the Java program example above. Then, using the add technique and the multiply method, respectively, we conduct addition and multiplication operations. The sum and product variables, which are presented on the console, contain the results.
The Java programming language’s BigInteger class has a variety of methods for carrying out different operations, including subtraction, division, exponentiation, modular arithmetic, and more. Additionally, it provides conversion between text representations and other numeric kinds.
The outcome of an operation is always a new BigInteger object since BigInteger objects are immutable (their values cannot be modified once they have been created).