Structure Declaration and definition
Use of Structure Data Type (Structure) Members In C Programming, different types of program data type variables are grouped together and processed under a single structure member name. Structures in C language provide you the features to create and process complex data type programs. Remember that any C program structure can have different types of different data type variable members.
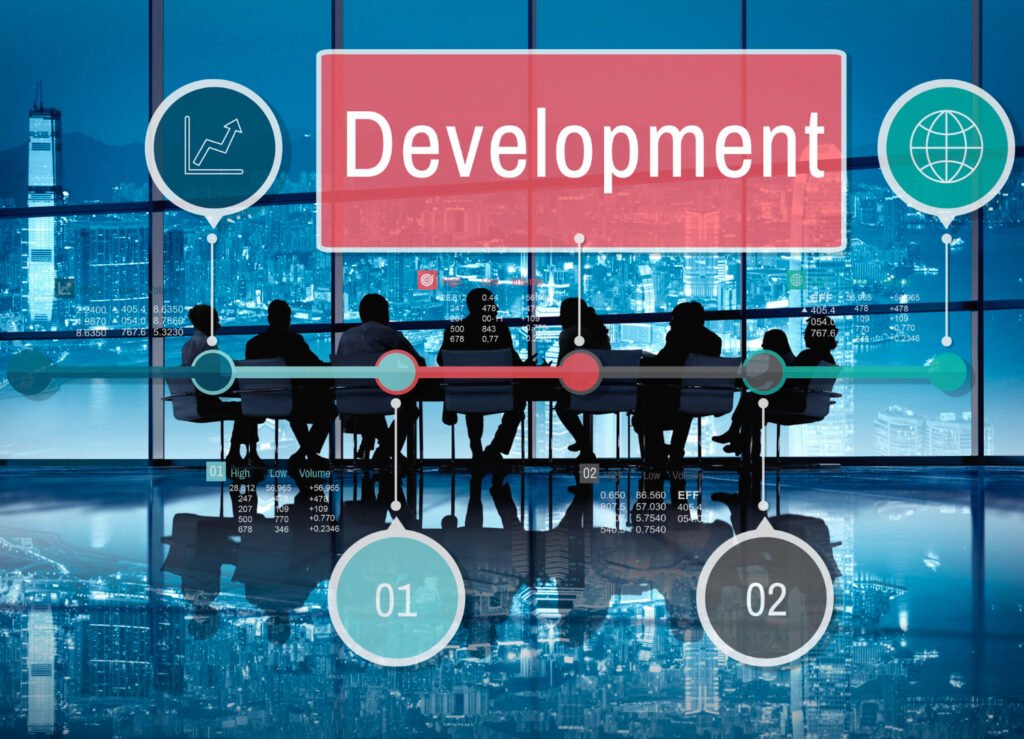
So let us declare and process structure member data type in C Programming.
Structure Declaration in C Programs.
To declare structure data type in C program, you can use the structure keyword present in the C library. After declaring the structure keyword, give the name of the program structure to be created and declare different structure data type variable members in curly braces {}. Remember that you can call and process different data type variables in a structure in a single structure member group. Where different data types declared in the structure have their individual role.
struct employee {
char emp_name[50];
int emp_age;
float emp_salary;
};
In the above structure example.
Employee name is the structure declaration of tag.
Within the {} curly braces of structure data type, emp_name[50], emp_age and emp_salary, etc. are the members of data type structure of type char, int, float.
Where emp_name[50] is a (string) array variable data type nature for employee.
Employee age data type in emp_age structure is integer data type variable value holder.
emp_salary is a floating data type variable structure member to store the salary of employee.
Structure declaration and initialization in C.
Remember that after declaring a structure in a C program, you can declare your existing structure data type variable as a structure member with individual different data types. You can initialize these structure member definitions based on the requirement.
struct employee employee0; // hare employee name Variable define with name type ’employee0′ of ‘struct employee
// you can see how to Initializing individual structure members data type
strcpy(employee0.emp_name, “David”);
employee0.emp_age = 45;
employee0.emp_salary = 1000.45;
// Accessing each and individual structure members
printf(“\n employee Name – %s”, employee0.emp_name);
printf(“\n employee Age – %d”, employee0.emp_age);
printf(“\n employee salary – %.f”, employee0.emp_salary);
Declare nested structures in C.
Sometimes in a C program, there may be a need to create more than one complex program structure data type variable. When more than one individual structure can be declared in an already existing structure or a structure can be declared and processed within another structure. Then such a structure is called a nested structure.
Nested Structure Example in C Programming.
struct emp_id {
char emp_department[40]
int emp_id;
float emp_height;
};
struct employee {
char emp_name[50];
int emp_age;
float emp_salary;
};
struct employee employee0;
In the above structure declaration example, you have declared a structure named employee, in this, a nested structure named emp_id is declared as a nested structure.
Typedef Data Type for Structures in C Programs.
In a C program, you can use typedef user defined data type along with structure data type. Remember that typeddef is a user defined data type member in C program. Like structure, you can process different data type variable members in typedef also.
typedef struct {
char course_nam[50];
int course_duration;
float course_price;
} course;
Now you can directly declare data variable members of course typedef data type without using struct keyword.
course coursename ;
coursename.course_duration = 2;
strcpy(coursename.course_name, “c programming”);
coursename.course_price = 1495.50;
Basic structure initialization in C.
In C program, structure data type is declared as structure. After this, based on program requirement, structure member is declared as individual data type variable in nature. Every structure member is initialized at this time.
struct employee {
char emp_name[50];
int emp_age;
float emp_salary;
};
struct employee employee0 = {
.emp_name = “Robert mathew”,
.emp_age = 45,
.emp_salary = 999.45
};
Some important facts about structure data type.
Structure declaration in C – After strcut keyword, define structure member data type and variable tag.
what is structure – Structure in C is a user defined data type in which different data type members are declared and processed.
what is nested structure – Multiple structure declaration method in a structure is called nested structure.
typedef data type – Typedef data type can be declared in a structure.
struct initialization – You can initialize a structure by specifying different data types in the structure.