String manipulation functions (strcpy, strcat, strlen, etc.)
String Manipulation Built-in string functions are used in C programming language to perform various types of string operations on string text or paragraphs. You can apply many string operations in C program by declaring character string as array such as copying a string, joining two different strings, comparing two different strings and finding the length of a string etc.
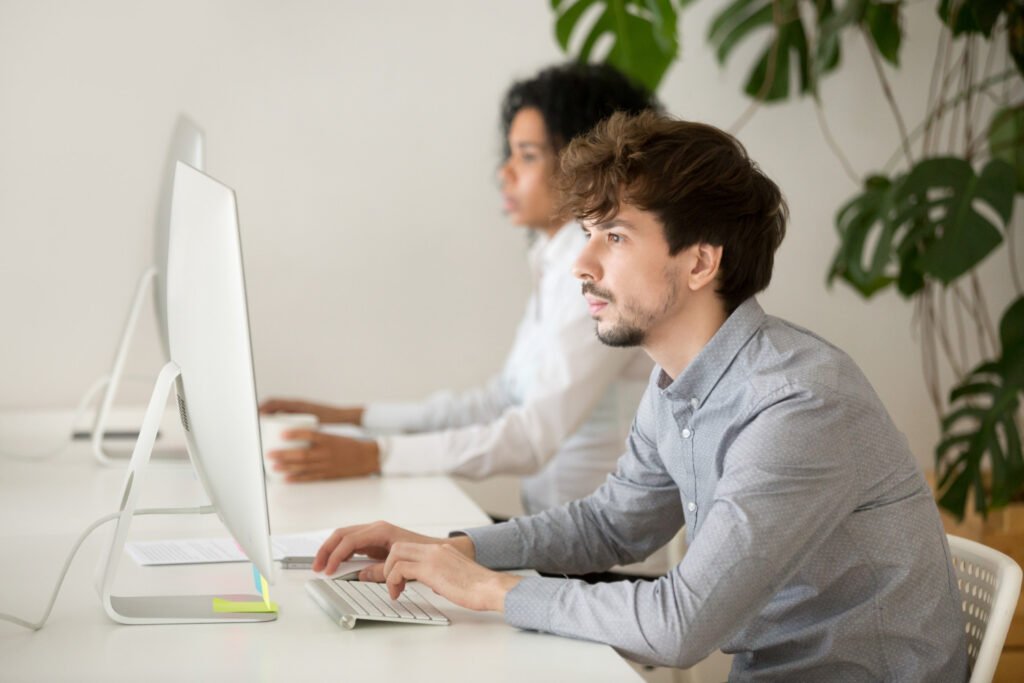
So let’s know the commonly used string operations in C programming.
strcpy (string copy) function in C.
#include <string.h> ////string.h header file must be added, otherwise the program will not perform any string operations.
char *strcpy(char *targetstr, const char *srcstr);
strcpy function – strcpy function in C program copies the src string text up to string (null terminator ‘\0’) in array string to target string text. In general language string copy function copies source string to destination string.
strcpy function syntax.
destination string – the source string to be copied into the destination string array.
string source – the original string to be copied into the destination string.
strcpy function example in C.
#include <stdio.h>
#include <string.h>
int main() {
char targetstr[50];
const char *srcstr = “welcome to vcanhelpsu”;
strcpy(targetstr, srcstr);
printf(“\n the source string Copy into target string – %s”, targetstr); // it copy source string into target string
return 0;
}
strcat (string concatenate) function in C.
#include <string.h>
char *strcat(char *dest, const char *src);
String concatenate function in C program concatenates target string at the end of src string (null terminator ‘\0’).
concatenate function syntax.
target string – target string is the pointer location of character array which has enough space to hold the result of concatenated string.
src string – pointer location of source string to be appended at the end of old string.
String concatenate function example in C.
#include <stdio.h>
#include <string.h>
int main() {
char targetstr[50] = “C”;
const char *srcstr = ” programming”;
strcat(targetstr, srcstr);
printf(“\n Connected source string in source string – %s”, targetstr); // now this add target string into the end of the source string
return 0;
}
strlen(string length) function in C language.
#include <string.h>
size_t strlen(const char *str);
strln function in C program displays the length of string str up to any input character array text null-terminated point or number of character string numeric value where the string ends, excluding the null terminator.
String Length Syntax in C Program.
array string – C program calculates the length of a given string number of character numeric value up to the null-terminated location of the declared character array string.
strln function program example in C.
#include <stdio.h>
#include <string.h>
int main() {
const char *str = “welcome to vcanhelpsu”;
st_length = strlen(str);
printf(“\n th String length is – %zu”, st_length); // see the actual string length in c program
return 0;
}
strcmp (string comparison) function in C language.
#include <string.h>
int strcmp(const char *str1, const char *str2);
strcmp string comparison function in C program compares two different character string arrays, where the first string string1 is compared with string 2.
string comparison function syntax.
string1, string2 – Here string first is compared with string second, and the string to be compared is a pointer address for a null pointer string to indicate the difference.
output string.
If the first string in the current program is less than, equal to or greater than the second string, then it displays the string comparison value which is less than, equal to or greater than zero.
String comparison program example in C.
#include <stdio.h>
#include <string.h>
int main() {
const char *text1 = “java”;
const char *text2 = “python”;
int output = strcmp(text1, text2); if (output < 0) {
printf(“\n %s string is less than %s -“, text1, text2);
} else if (output > 0) {
printf(“\n %s string is greater than %s -“, text1, text2);
} else {
printf(“\n %s the both text string is equal to %s -“, text1, text2);
}
return 0;
}