Structure pointers
Using pointer operators for structure data type members allows you to efficiently manipulate structure data type members within the declared structure and to access structure members from memory address locations, especially when dynamic memory allocation is needed for a structure or passing a function to a structure.
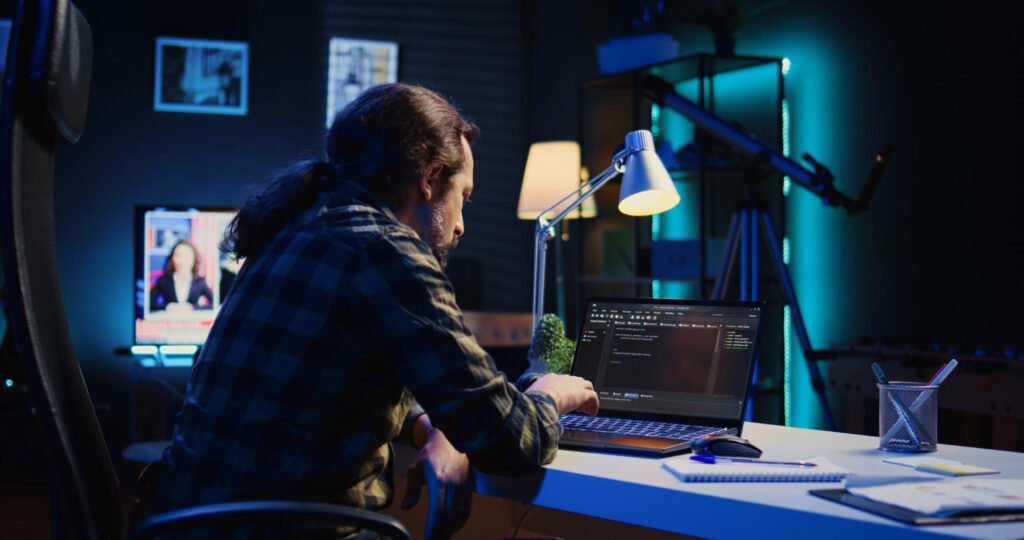
So let’s use structure to pointer members in C program.
Declare and initialize structure pointers in C program.
To declare a pointer to a structure data type variable, you use the structure keyword followed by the structure name and then use the (*) pointer/initialize operator to declare this structure as a pointer.
Pointer to structure example.
#include <stdio.h>
#include <stdlib.h> // for malloc function to declare dynamic memory in structure
// let Define a employee name structure
struct employee {
char name[50];
int age;
float salary;
int main() {
// let Declare a pointer to an employee struct data type
struct employee *employeePtr;
// let Allocate memory for an employee struct with malloc function
employeePtr = (struct employee *) malloc(sizeof(struct employee));
if (employeePtr == NULL) {
fprintf(stderr, “\n employee structure Memory allocation denied”);
return 1;
}
// let Initialize employee structure members through pointer variable
strcpy(employeePtr->name, “david mathew”);
employeePtr->age = 60;
employeePtr->salary = 999.34 ;
// lets Access and print employee structure members through pointer operator
printf(“\n employee Name – %s”, employeePtr->name);
printf(“\n employee Name Age – %d”, employeePtr->age);
printf(“\n employee Name salary – %.f”, employeePtr->salary);
// let Free allocated memory when employee structure members done
free(employeePtr);
return 0;
}
Explanation of the above program.
C Program Structure Definition (struct Employee).
Here an employee structure data type is defined with three members named employee. In which employee structure data type has employee name (character array), employee age (integer), and employee salary (floating) data type.
Pointer declaration (struct employee *employeePtr):
Here the structure employeePtr is declared as a pointer to the structure employee.
Memory allocation in struct (malloc(sizeof(struct employee))):
Dynamically allocates memory for structure employee using malloc function in the existing structure. Where size of existing structure is defined using sizeof(struct employee).
Error handling in struct.
Here in employee structure it checks whether memory allocation (malloc) function operation was successful or not. If employeePTR is zero, then memory allocation failed, and an error information is displayed.
Accessing structure members in C.
Structure members are accessed and initialized using arrow (->) structure pointer operator through employeePTR.
Printing structure members in C program.
Variable value stored in structure is printed through employeePTR structure.
Freeing memory in C struct (free(Employee ptr)).
Use of free() in C structure is to free the program allotted memory by using free function to prevent memory leaks.
C structure pointers additional use.
Passing structure pointer to function – Remember that C structure pointers operator is usually used to pass structure data type variable value from memory address to function efficiently.
void printemployee(struct employee *ptr) {
printf(“\n employee Name – %s”, ptr->name);
printf(“\n employee Age – %d”, ptr->age);
printf(“\n employee salary – %.f”, ptr->salary);
}
/ employee pointer use.
printemployee(employeePtr);
Structure Dynamic Memory Allocation – Structure pointers are used to process dynamic memory allocation (malloc(), calloc(), realloc()) functions to manage memory efficiently in C structure programs.
Nested Structures – Pointers are used with nested structures to access and manipulate structure data at different levels of hierarchy.
Key points to remember for pointer to structure data type.
Arrow (->) operator is used with pointer to structure pointers to access structure members.
Use malloc() memory allocation and free() memory free function appropriately when working with structure pointers to avoid memory leaks in pointer to structure programs.
Understanding how to use pointer to structure effectively in C programs is a fundamental concept in C programming. Especially where you need to use pointer operators in C++ programs is when you need to manage complex data structures and dynamically manage structure members variable memory.