Pointer arithmetic
Pointer arithmetic operation in C language is a basic fundamental programming concept in languages like C and C++. In which direct C program variable memory management features are available. It allows the C programmer to manipulate the memory addresses stored in pointers to efficiently navigate and manage array and other data structure operations.
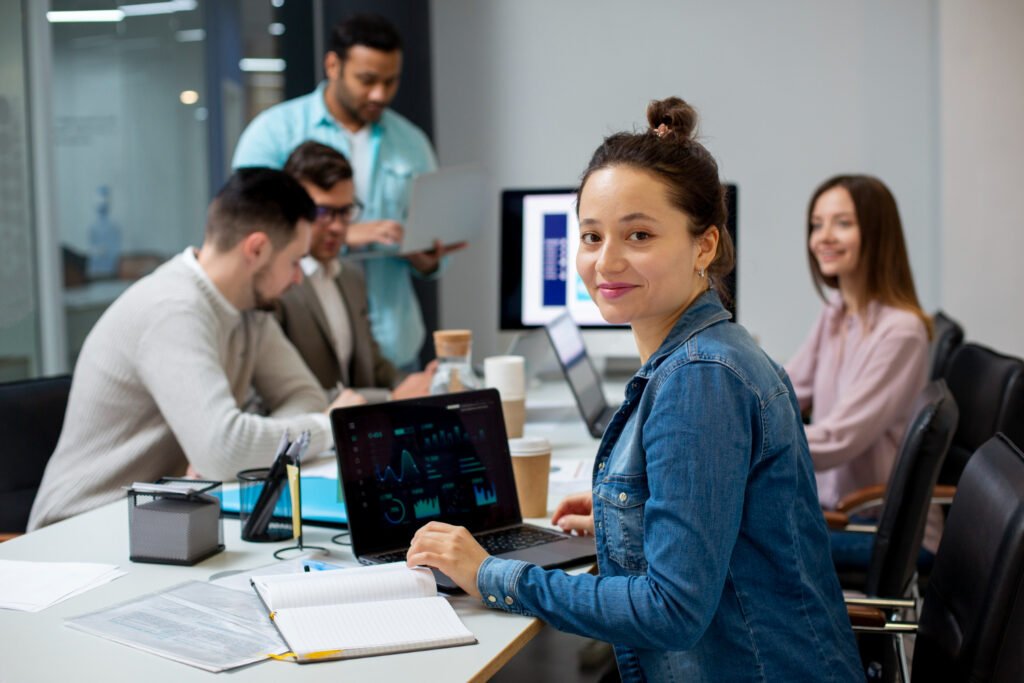
So let’s know what is pointer arithmetic in C.
Pointer Definition and Dereferencing in C.
A pointer in C language is a memory variable and its storage address. Which stores the memory address location of a variable declared in another program.
Pointer dereferencing in C language means knowing the value stored at the memory address stored by the pointer variable declared in the program.
int p = 9;
int *ptr = &p; // Here ‘ptr’ variable now holds the address of ‘p’
int info = *ptr; // Here the variable ‘info’ now holds the value 10.
Pointer Addition and Subtraction Operations in C.
Remember that in C language adding an integer to a pointer advances it not by bytes but by the number of stored array elements.
Subtracting an integer value from a pointer to an array decreases it by the number of array elements.
int arrinfo[] = {9, 5, 8, 3, 2};
int *ptr = arrinfo; // ‘arrinfo’ indicates the first stored element of the array
ptr++; // Now ptr indicates the second stored element ‘5’ (arrinfo[1])
ptr–; // Here now ptr indicates the second stored array element ‘9’ (arrinfo[0])
ptr += 3; // Now points to the array element at ‘2’ (arrinfo[4])
Pointer Difference in C.
Pointer Difference in C is used to find the values of array variable elements by subtracting one pointer from another.
int *start = arrinfo; // This indicates the first array index element arrinfo[0].
int *end = arrinfo + 4; // This indicates the last 4th array element in arrinfo[4].
int distance = end – start; // This indicates that the ‘distance’ between the first and last array element index is 4.
Comparison of Pointers in C.
In C language pointers can be compared between pointer variables using relational operators like ==, !=, <, >, etc.
Remember that pointer comparisons are valid only when the pointers are displayed or stored in the same array element or memory block element.
if (start < end) {
// when it find condition true, it display result
}
Pointer Arithmetic on Different Types in C.
If you want to perform pointer arithmetic operation in a C program. Then the result of pointer arithmetic may vary depending on the data type used for the pointer.
If you increment a pointer in char data type, it moves forward by 1 character byte. Whereas if you increment a pointer in int integer data type, it moves forward by 4 bytes.
char *charptrinfo = (char *) array; // Declaring ‘arr’ as a char character array.
int *intptrinfo = array; // Declaring ‘array’ as an integer array.
charptrinfo += 1; // It moves forward by 1 byte in the memory
intptrinfo += 1; // moves forward by 4 bytes (or sizeof(int) bytes)